AppleInsider could earn an affiliate fee on purchases made by means of hyperlinks on our website.
In a earlier article, we checked out connecting varied sorts of Arduino {hardware} to your Mac. Here is tips on how to get began programming on them to create your individual tasks.
Getting began
So as to program your Arduino, you want the Arduino IDE (Built-in Growth Surroundings) from the arduino.cc web site. We talked about tips on how to obtain and set up the IDE within the earlier article however we’ll reiterate it right here:
In your Mac, go to arduino.cc, click on on “Software program”, then underneath the Obtain Choices part, click on the hyperlink for the Mac model for both Intel or Apple Silicon.
Word that until you wish to discover the present growth model, you do not need the hyperlink underneath the part “Nightly Builds”. Nightly builds could also be unstable and comprise bugs.
As soon as you have downloaded the IDE, check with the earlier article for particulars about tips on how to join and arrange your Arduino in your Mac within the IDE. You want a connection earlier than you may add code from the IDE to your Arduino.
Fundamentals
You solely want rudimentary programming expertise to program your Arduino. You should utilize Arduino’s C-like programming language, or Python, however we’ll solely use C within the examples under.
In Arduino applications, known as Sketches, you primarily write code to arrange your Arduino for a selected duties or duties, then run a steady loop which will get known as repeatedly by the Arduino microcontroller as soon as your Sketch is uploaded to your Arduino system. The loop normally waits for enter from sensors or customers, and sends management alerts again to sensors and gadgets to show some type of output.
The loop code may also hook up with, and work together with, the web.
You can too set up third-party libraries which assist varied sensor gadgets and shields utilizing the Library Supervisor within the IDE. To entry the Library Supervisor, create or open a sketch window, and click on the icon on the left aspect which appears like a set of books:
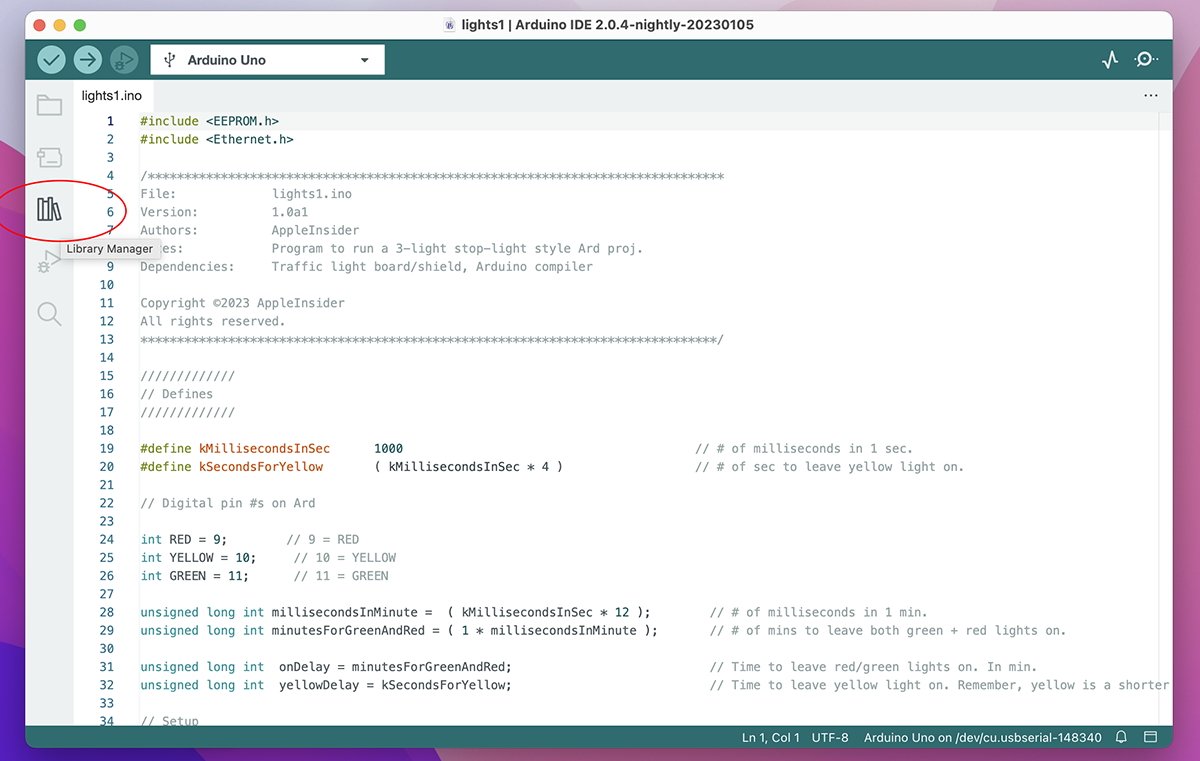
A pane will seem and on the high, you may browse obtainable libraries by Kind and Subject by clicking both of the 2 popup menus:
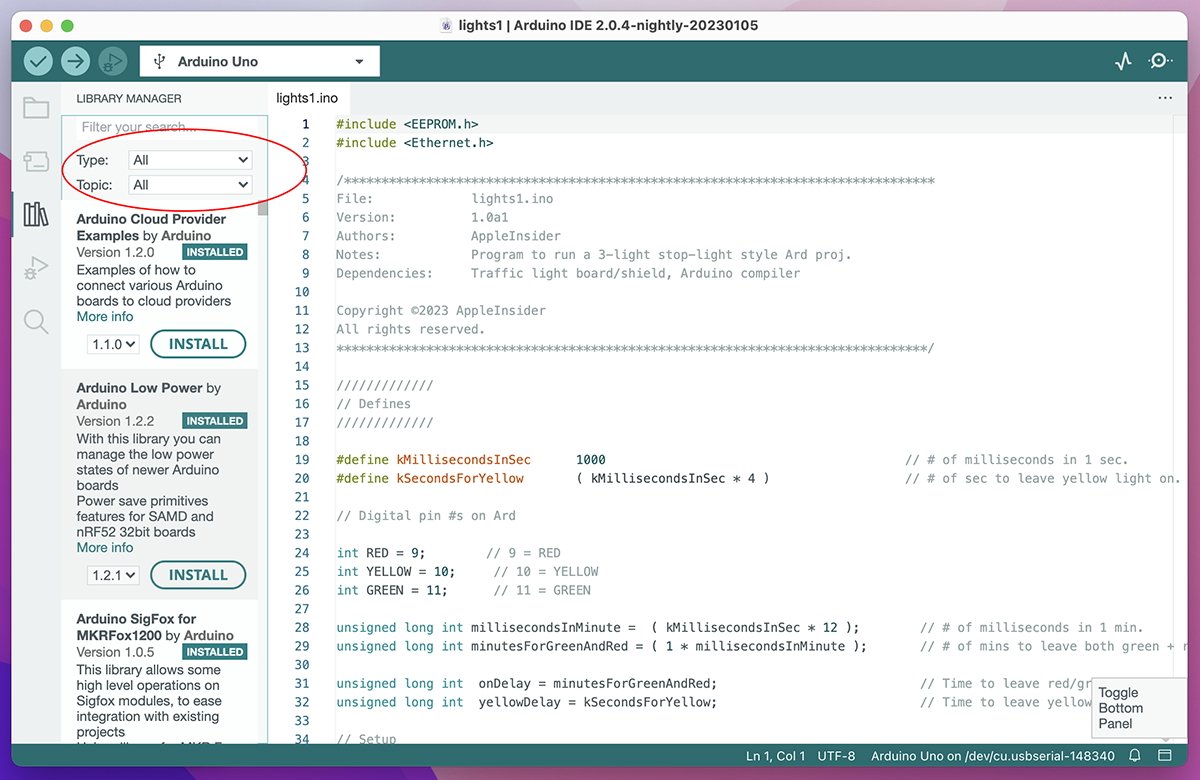
You can too verify for put in library updates by choosing “Updatable” from the “Kind:” menu.
The Library Supervisor pane enables you to obtain official and third-party libraries. Libraries are code bundles that add a selected performance or system assist to the IDE.
For instance, should you use a specific model of a non-generic sensor, you may have to obtain its library and set up it first.
You possibly can view totally different classes of libraries by clicking the “Subject” popup menu on the high of the Library Supervisor window.
Most Arduino Sketches are easy and brief and are saved in a “Sketchbook” folder specified within the Arduino IDE Settings window. You possibly can change the place the IDE shops sketches from right here by clicking the Browse button subsequent to “Sketchbook location”:
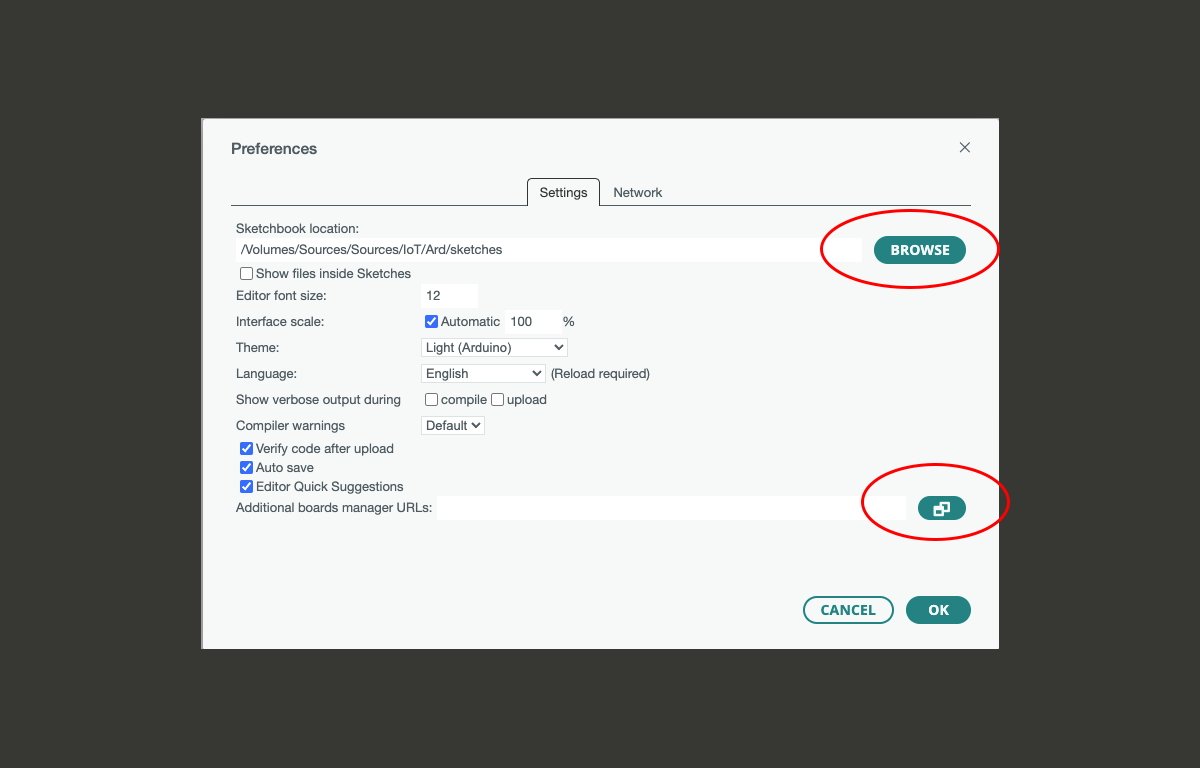
Sketches have a file extension of .ino, which stands for “innovation”.
You can too change textual content editor and compiler settings right here. When you’ve got an unsupported Arduino, you may add its board assist file or URL by clicking the small icon within the decrease proper of the window, one which appears like a stack of paperwork.
Click on on the “Click on for an inventory of unofficial board assist URLs” textual content within the Extra Boards Supervisor URLs window to view the huge array of boards supported on Arduino’s GitHub.
The Arduino IDE gives a pattern sketch with a easy program define in a textual content window whenever you first open it.
There are additionally an enormous array of code samples underneath the File->Examples submenu merchandise. Samples are organized by “Constructed-In” and “UNO Examples” within the menu. Customized-installed libraries can also present examples.
A primary instance sketch
In our first instance, we’ll use the built-in Blink instance. To open it, choose File->Examples->0.1Basics->Blink submenu merchandise.
After a couple of seconds, a brand new editor window will open with the Blink instance. Make sure that your Arduino board and port are chosen from the connection popup menu on the high of the IDE’s editor window.
Blink does one factor — it blinks a built-in LED on the Arduino. There is a description of the pattern within the remark on the high of the editor window and on-line.
In code, feedback are notes programmers go away to explain what code is doing. Feedback are ignored throughout compilation.
The IDE editor window makes use of C-style feedback: the compiler will ignore something bracketed inside /* and */. Single-line feedback begin with // however have to be on one line solely. Something after the // is ignored.
Once you click on the big Confirm button with the checkmark icon within the editor’s higher left nook, the IDE will compile the code in that window.
Throughout compilation, an Output pane will seem on the backside of the editor window in black exhibiting progress. If there are not any errors, you may see messages equivalent to:
"Sketch makes use of 924 bytes (2%) of program space for storing. Most is 32256 bytes.
World variables use 9 bytes (0%) of dynamic reminiscence, leaving 2039 bytes for native variables. Most is 2048 bytes."
If there are errors, they’ll seem in purple textual content and you will have to repair your code till there are not any extra errors. Some errors are usually not crucial, and your sketch will nonetheless run with them, however different errors can stop your sketch from operating in any respect.
You possibly can clear the Output messages by clicking the small icon within the higher proper nook of the Output pane. You possibly can cover the Output pane completely by clicking the small sq. icon within the decrease proper nook of the editor window.
Once you click on the Add button (the one with a big proper arrow icon) subsequent to the Confirm button, the IDE uploads the compiled binary program into the Arduino on the port you specified. The Arduino microcontroller takes over from there and executes your code on the Arduino.
Throughout add, in case your Arduino has an RX (Obtain) LED built-in, it is best to see it flash quickly because it receives the sketch information.
In case your Sketch could not be uploaded for any purpose, the Output pane will checklist an outline and why.
Just a few phrases about C-based languages
C is the language of working programs. Some early Mac apps within the late Nineteen Eighties and the Nineteen Nineties had been written in C or considered one of its later variants: C++.
The Arduino IDE programming language relies on C-like syntax.
In most C-like languages, all code traces finish with a “;” — with out the semicolon, the code will not compile and you will get an error.
Most C-based languages additionally use predefined code textual content information known as headers, which normally have a “.h” file extension.
Consider a .h file as a predefined set of code that describes how features are to be accessed, known as prototypes. Every prototype defines a perform identify, parameters to be handed to the perform (inside parenthesis), and a return sort that’s despatched again from the perform when it exits.
In the event you name (entry) any library or built-in features in your Sketch code, the way you name every perform should match its prototype outlined in a .h file someplace. Libraries work the identical method.
For instance, if a prototype says a perform should take two enter parameters (within the parenthesis), and a sure sort of return worth (listed earlier than the perform identify), then you need to name it in exactly the identical method. Anything will throw an error throughout compilation.
Header information may also comprise a C-style assemble known as a ‘outline.’ A outline creates a label as one other code expression, equivalent to a quantity, textual content (a string in C), a calculation, or another perform.
To create a outline, you utilize the #outline C preprocessor directive. For instance:
This code defines the label ‘FALLING’ as the worth of two. Anyplace you utilize FALLING in your code, the quantity 2 will probably be substituted at compile time. #defines can get fairly advanced however could make your code shorter and extra readable.
The Arduino IDE makes use of #defines to outline issues like I/O pin numbers, modes, and different issues.
You can too create your individual headers and #defines.
Headers will be included in different information – in different .h information, or in Sketches themselves. The contents every included .h file get inserted at compile time into the highest of any information they’re included in.
To insert a header into one other file, use the #embrace C directive. For instance on the high of Arduino.h you may see:
Which incorporates one other header file known as “binary.h” into the highest of Arduino.h at compile time.
In the event you take a look at the screenshot proven above you may see two included .h information within the instance Sketch:
All this will appear complicated at first, but it surely’s truly fairly easy: you set perform prototypes and #defines in .h information to allow them to be utilized in many different information. You then #embrace them in different information and the compiler inserts them the place indicated throughout compilation. Straightforward.
Organizing definitions into seperate headers permits code reuse.
Simply consider .h information as definitions, and your Sketch information as applications that use them.
Fashionable programming languages equivalent to Apple’s Swift and Microsoft’s C# have executed away with header information, within the curiosity of simplicity.
Again to the Blink instance
Within the Blink pattern’s setup() perform, there may be one line of code:
pinMode(LED_BUILTIN, OUTPUT);
Each LED_BUILTIN and OUTPUT are #defines outlined by Arduino headers. The primary Arduino header file is called Arduino.h, as we noticed above.
You possibly can maintain down the Command key in your Mac keyboard and double-click any #outline in an editor window to leap to its definition within the corresponding .h file the place it is outlined. A brand new tab will open in the identical editor window displaying the matching .h file.
LED_BUILTIN is outlined as “13” in pins_arduino.h:
This means digital pin 13 on the Arduino’s pins connector (additionally known as a header).
However on the identical time LED_BUILTIN tells the Arduino to make use of the precise built-in LED on the Arduino circuit board itself. In the event you join a jumper wire to pin D13 on the Arduino header, then join it to an LED on a breadboard, it should blink that LED additionally.
pinMode’ is a built-in Arduino perform that units how a given I/O pin on the Arduino behaves – both enter or output. On this case, we’re telling the Arduino to make use of pin LED_BUILTIN (13) as an output pin.
Since pinMode()’s prototype within the wiring_digital.h header has a return sort of ‘void’, the perform would not return any worth. ‘void’ is a C information sort that means ‘nothing’. All C features having a ‘void’ return sort return nothing.
When the compiled Blink sketch runs in your Arduino, it runs setup() first, establishing the {hardware}, then it runs loop() again and again ceaselessly. Within the Blink instance, loop() merely turns the pin on and off with a delay of 1 second between every:
digitalWrite(LED_BUILTIN, HIGH); // flip the LED on (HIGH is the voltage degree)
delay(1000);
digitalWrite(LED_BUILTIN, LOW); // flip the LED off by making the voltage LOW
delay(1000);
“Excessive” and “Low in electrical engineering phrases merely imply “on” and “off”.
digitalWrite() is a built-in Arduino perform that merely turns the digital U/O pin specified on or off – on this case no matter is hooked up Arduino pin D13, or LED_BUILTIN.
delay() is a delay perform that halts additional processing till the desired interval has elapsed. delay() takes a single time worth, in milliseconds, with a price of ‘1000’ being one second.
Go forward and click on the Add button within the Blink Sketch IDE window.
That is it. You have now compiled and run your first sketch. If every little thing labored, you will note the built-in LED in your Arduino blink on and off.
Most shields even have a built-in LED on them which does the identical factor because the built-in one on the Arduino.
A breadboard instance
Now that you have seen the Blink instance in motion, we’ll do the identical factor – however this time we’ll make an exterior LED on a breadboard blink on the identical time. For this instance you may want:
- A breadboard
- 2 jumper wires
- 1 3.3V LED
- 1 220 Ohm resistor
First, plug two jumper wires into the “D13” or “13” and “GND” holes on the corresponding Arduino headers.
Plug the opposite finish of the “D13” wire wherever into row one within the inside of the breadboard (any place besides within the “+” and “-” energy rails on the outer edges).
Subsequent, set up the LED onto the breadboard so the lengthy (+) leg of the LED is in the identical horizontal row because the “D13” wire you simply put in.
Flip the LED sideways so the brief leg is inserted right into a gap in direction of the lengthy finish of the breadboard about three holes away.
Subsequent, insert a 220 Ohm resistor into the identical horizontal row because the brief LED’s leg, however in direction of the facility rail on the other aspect of the board. Throughout the middle of the board works completely.
Insert the opposite finish of the resistor into one other gap in the identical route about three holes away.
It’s best to now have the “D13” wire, LED, and resistor in a sample considerably within the form of a stretched-out “Z” (see picture under).
On the different aspect of the breadboard within the final or second to final gap earlier than the facility rail, insert the opposite finish of the “GND” jumper wire. The ultimate meeting ought to look one thing like this:
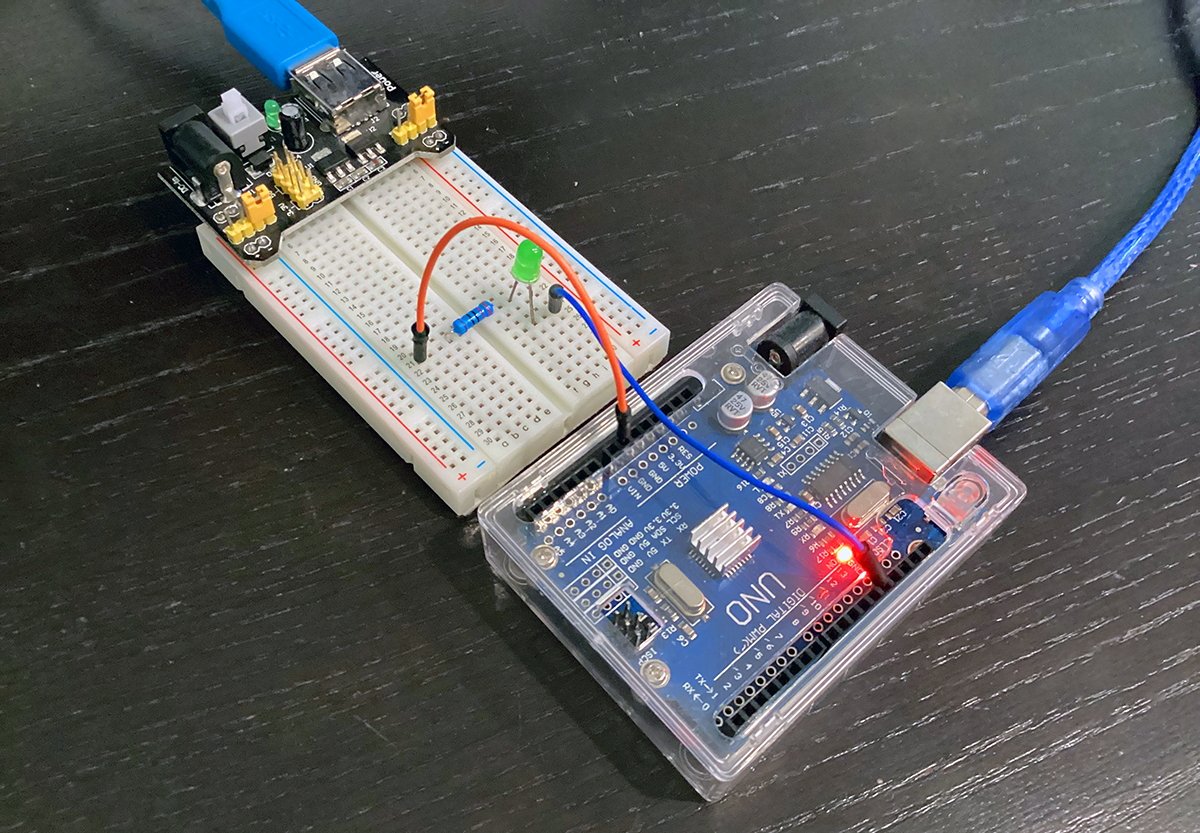
Keep in mind there’s a grid of metallic rails underneath all of the holes on the breadboard. Irrespective of which method parts are oriented on the breadboard, they need to all the time kind connections between parts, the Arduino, and with jumper wires to finish the circuit.
In the event you already uploaded the Blink instance to your Arduino, it is best to see the LED begin to blink. Each the LED on the Ardunio and the one on the breadboard ought to blink in unison. If not, return and verify all of your connections once more.
Word that some digital parts equivalent to LEDs are polar: present can solely circulation by means of them accurately in a single route. Different parts equivalent to most resistors are non-polar: present can circulation the identical by means of them in both route.
You possibly can mess around with the timing worth handed to the delay() perform to hurry up or decelerate the blink price of the LEDs. Attempt big and tiny values and watch what occurs.
By including extra digitalWrite() and delay() statements to your Sketch you may alter the blink sample: for instance, you may make the LEDs blink Morse Code.
Last instance: a visitors mild simulator
Now that you have seen tips on how to blink your Arduino’s LED, we’ll use one closing, barely extra advanced instance: we’ll use a third-party UNO breakout board and an exterior visitors mild sensor board to simulate a three-color visitors cease mild.
Alternately, you will discover a temporized one with a countdown timer LCD additionally on PCBWay.
In our instance, we’ll use the only three-light sensor and make it change colours at transient intervals, identical to an actual visitors mild.
These visitors mild boards normally have three or 4 pins: one for every coloured LED, and one GND. The code is smilier to Blink, besides that you just flip all of the lights off besides one, wait utilizing delay(), then flip that one off, and the subsequent one on, in sequence.
First, we’ll join our visitors mild sensor to a generic breakout protect we now have put in on our Arduino UNO: breakout shields are shields containing banks of analog and digital pins, GND pins, Bluetooth, and serial cable connectors.
Our visitors mild sensor has 4 pins: R, G, Y, and GND. We’ll join digital pins 9, 10, and 11 to R, G, and Y respectively, and the fourth pin, GND to a GND pin on our protect.
Now open the Arduino IDE and begin a brand new Sketch. First, we’ll outline some issues we’ll want on the high of the Sketch above setup().
First, we outline what number of milliseconds are in a second so we are able to specify what number of seconds to move to delay();
#outline kMillisecondsInSec 1000
Subsequent, we outline what number of seconds we would like the yellow mild to be on for:
#outline kSecondsForYellow ( kMillisecondsInSec * 4 )
Then we outline which three pins we wish to use for purple, yellow, and inexperienced on the Arduino. As an alternative of utilizing defines, we assign the pin values every to a world variable, on this case variables of sort int (which is outlined by C):
int RED = 9;
int YELLOW = 10;
int GREEN = 11;
Consider a variable as a named container whose contents (worth) you may change everytime you need. Variables even have a sort in an effort to specify what sort of values they’ll maintain. World variables will be accessed from wherever in a program.
Variables declared inside features are known as native variables and might solely be used inside one perform. That is known as variable scope.
Subsequent, we outline world variables and assign calculations to them to simplify how we calculate seconds and milliseconds, and delay values for the inexperienced, purple, and yellow lights in seconds. On this case we use a variable sort known as ‘unsigned lengthy int’, that are like ints, however can maintain bigger values:
unsigned lengthy int millisecondsInMinute = ( kMillisecondsInSec * 12 ); // # of milliseconds in 1 min.
unsigned lengthy int minutesForGreenAndRed = ( 1 * millisecondsInMinute ); // variety of minutes to go away inexperienced, purple lights on.
unsigned lengthy int yellowDelay = kSecondsForYellow; // Time to go away yellow mild on. Yellow delay is shorter than purple/inexperienced.
Now in setup() we outline the pin modes for pins we outlined above, one every for purple, yellow, and inexperienced:
void setup( void )
{
pinMode( RED, OUTPUT );
pinMode( YELLOW, OUTPUT );
pinMode( GREEN, OUTPUT );
}
This tells the Arduino we’ll use these three pins for output values (on this case on or off).
In loop(), we begin by turning the purple/yellow lights off, the inexperienced mild on, then we look forward to onDelay seconds:
void loop( void )
{
// Inexperienced - Begin
digitalWrite( RED, LOW );
digitalWrite( YELLOW, LOW );
digitalWrite( GREEN, HIGH );
delay( onDelay );
After onDelay, we flip the inexperienced mild off, the yellow mild on, then look forward to yellowDelay seconds:
digitalWrite( GREEN, LOW );
digitalWrite( YELLOW, HIGH );
delay( yellowDelay );
After yellowDelay, we flip the yellow mild off, the purple mild on, and look forward to onDelay seconds:
// Crimson
digitalWrite( YELLOW, LOW );
digitalWrite( RED, HIGH );
delay( onDelay );
Lastly, after onDelay elapses, we flip the purple mild off, successfully resetting the simulation:
digitalWrite( RED, LOW );
}
The following time the loop runs, the identical sequence repeats, beginning with the inexperienced mild. This loop will run ceaselessly till stopped. We now have a working visitors mild simulator:
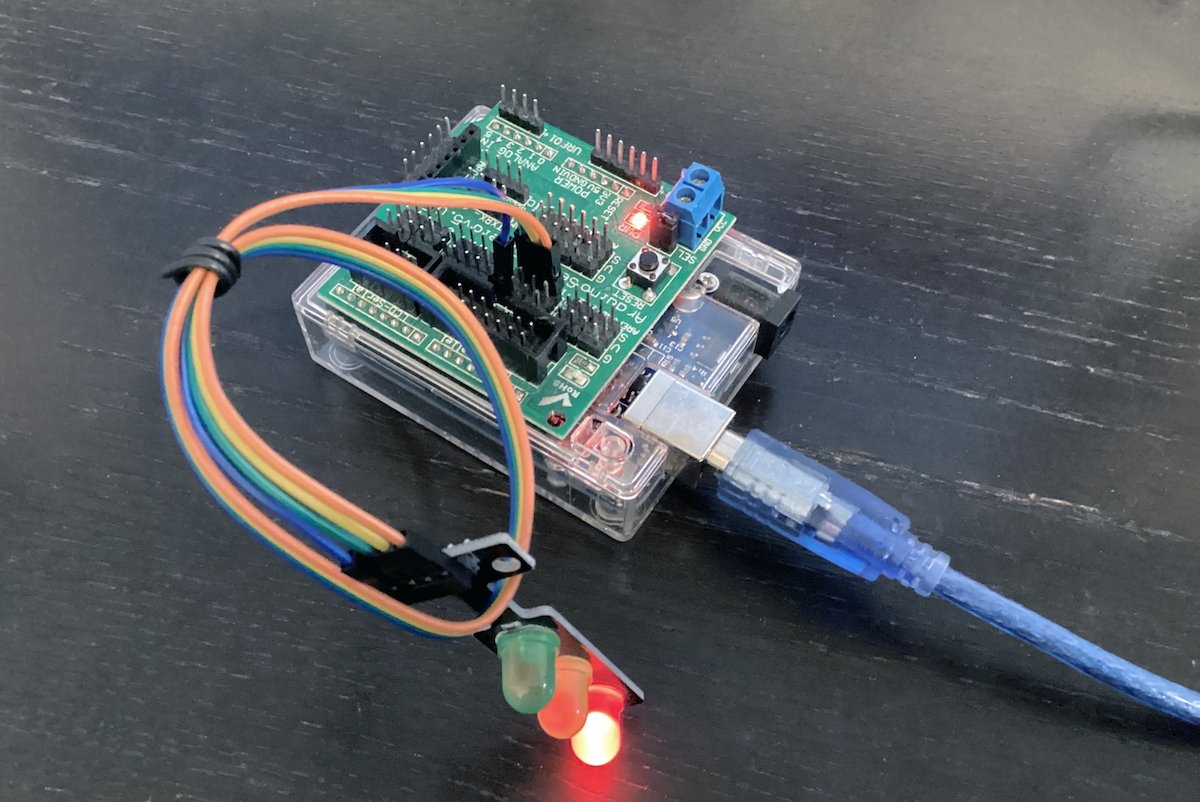
As soon as you have uploaded the Sketch to your Arduino, should you plug in an exterior energy provide to your Arduino’s DC barrel jack, and unplug its USB cable, the simulation will proceed to run.
This is without doubt one of the advantages of Arduino: when you program the microcontroller, it could run a program independently of a number laptop.
You can also make Arduinos do absolutely anything – act as sensors, show information, look forward to enter, make sounds, use cameras and ship pictures again to different gadgets, monitor situations, drive motors, and so forth.
I’ve posted the whole visitors mild Sketch. You possibly can obtain it and add it to your Arduino.
There are a couple of introductory books price studying for studying Arduino’s C-like language:
These easy examples ought to get you began programming Arduino. As you construct your programming expertise and achieve confidence, you may develop to greater examples.
In future articles, we’ll discover programming Arduino gadgets, shields, and busses equivalent to I2C and SPI, and creating advanced system environments.